What You'll Need | Initialise FlexPay Widget | Show Finance Offers | Eligibility Check | Start Checkout | Complete Checkout | Confirm Payment
4. Initialise FlexPay Widget
FlexPay Widget is a plugin to enable you to easily embed finance options in any page of your website as well as at checkout, where it can facilitate a finance-based purchase. In order to enable the plugin on a webpage, you must first initialise it.
The FlexPay Widget must be initialised before being used for any of the following steps:
- Promote Finance outside Checkout
- Show All Finance Offers outside Checkout
- Customer can optionally check their eligibility for FlexPay directly in the Widget
- Start Checkout
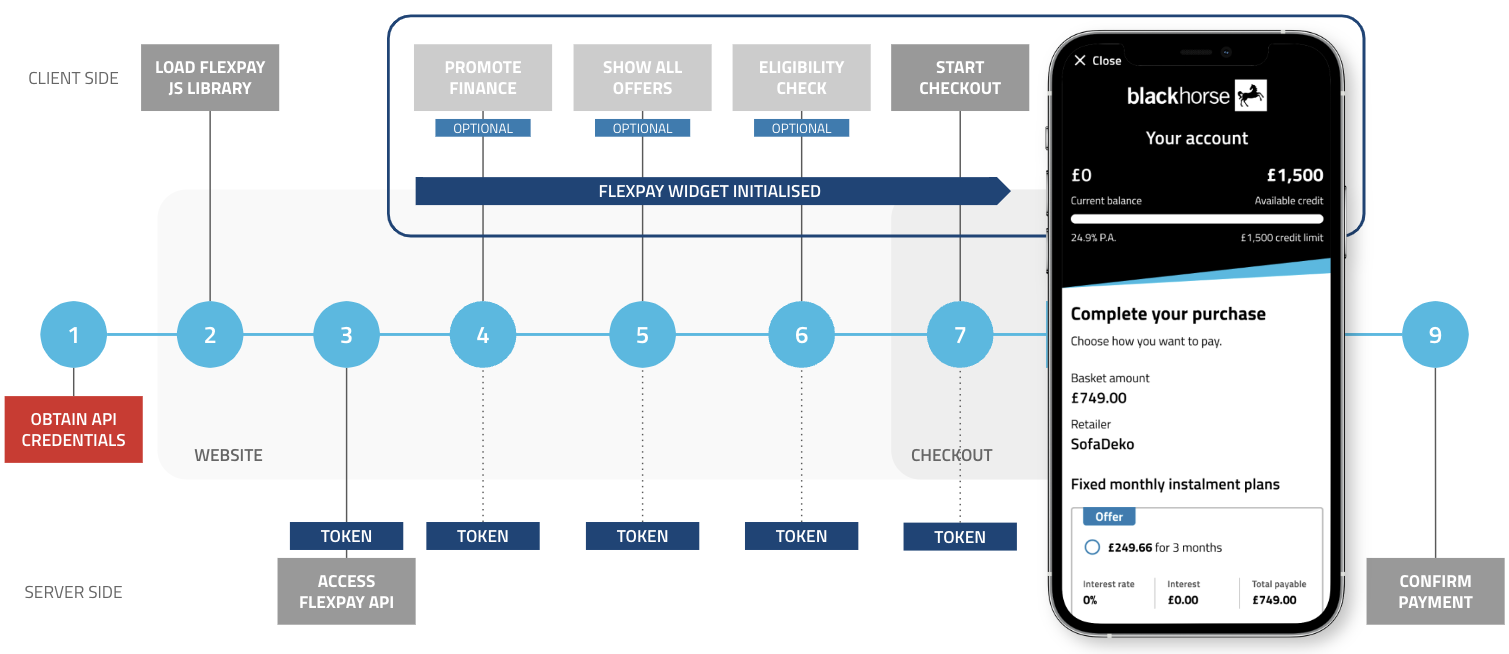
To initialise the FlexPay Widget please follow these steps, using the corresponding Access Token you obtained with your API credentials:
Obtain a JWT
Access the FlexPay API as illustrated in the prior step and then call the init endpoint in a machine to machine call to receive a JSON Web Token (JWT):
curl -X 'POST' \
'https://dummy-api.staging.host.com/init' \
-H 'accept: application/hal+json' \
-H 'Authorization: Bearer xeyJhbGciOiJSUzI1NiIsInR5cCI6IkpXVCIsImtpZCI6Imp6SXZmaU1HNkJqVEpUWGxkRlVwZSJ9.eyJpc3MiOiJodHRwczovL2Rla29wYXktbWVyY2hhbnQtZGV2LmV1LmF1dGgwLmNvbS8iLCJzdWIiOiJ2TDBac0VOOWo1TlQ0VlAzY0ZLQnAxakY5NHNtNUM4dkBjbGllbnRzIiwiYXVkIjoiaHR0cDovL2xvY2FsaG9zdDo4MDgxL2FwaS9jaGVja291dCIsImlhdCI6MTYxNjU5MTU4NywiZXhwIjoxNjE2Njc3OTg3LCJhenAiOiJ2TDBac0VOOWo1TlQ0VlAzY0ZLQnAxakY5NHNtNUM4diIsImd0eSI6ImNsaWVudC1jcmVkZW50aWFscyJ9.F-dzPkJVF0ub2kGiWn1As5PSMnIiqAriOhyux47t7lbNk0tD9Wu655JGHmjuAqqQ3uWyqXx9-feuYjv61xufgBfxH17OkIqBB9lVJzEONoVqF5uIzsnaTD2z0FTzcvOuH8RXzA-_q7vFZwvbboE9iZCFd-JN8vI_1jjWDUpdKFWzGk0-AmID9F7qgNnyC2WEb6DK4Ky5VttXPXcGfnIGD2ybOgkg1dOlBhzabJBT9jU_UQuBoKjqQoNqVm1ovhFJzzSWMsHqDIDOb7r-0zXRB33ka4_oKoVnVrvxL2hGblgC-2Jg6gfB7uuVPdbM5FIBFx8Cbt4T83KxXbpJy5hQbg' \
-d ''
The POST request returns the init_token.
FlexPay Widget
Initialise the FlexPay Widget via the window
object. This should be done in a closure to protect the scope. The function you pass to the flexpayWidget.init method must make a request to your server which in turn would request the init token from the FlexPay Public API. The return type of your function should be a Promise that resolves to the init token retrieved by your server. See below.
const yourFunction = () => {
return Promise.resolve(myInitToken);
}
const widget = window.flexpayWidget.init(yourFunction);
In this example, widget
is an instance of the flexpayWidget
Javascript object. This object provides functions enabling you to checkout and display or hide the widget UI.
Alternatively you may choose to initialise the Widget in a hidden state only so that the tile will not appear immediately on all (or some of) your website pages after it is initialised.
const yourFunction = () => {
return Promise.resolve(myInitToken);
}
const widget = window.flexpayWidget.init(yourFunction, {isVisible: false});
FlexPay Tile
Once the FlexPay Widget has been initialised you have the option to show or hide a floating tile on each webpage. This allows you to promote the availability of finance to customers browsing your site. Customers clicking on the tile will see finance offers and can check their eligibility.
Allowing customers to quickly see if they will qualify for finance from anywhere on your site prior to checkout, helps increase sales as well as your customers intended spending or product selection.
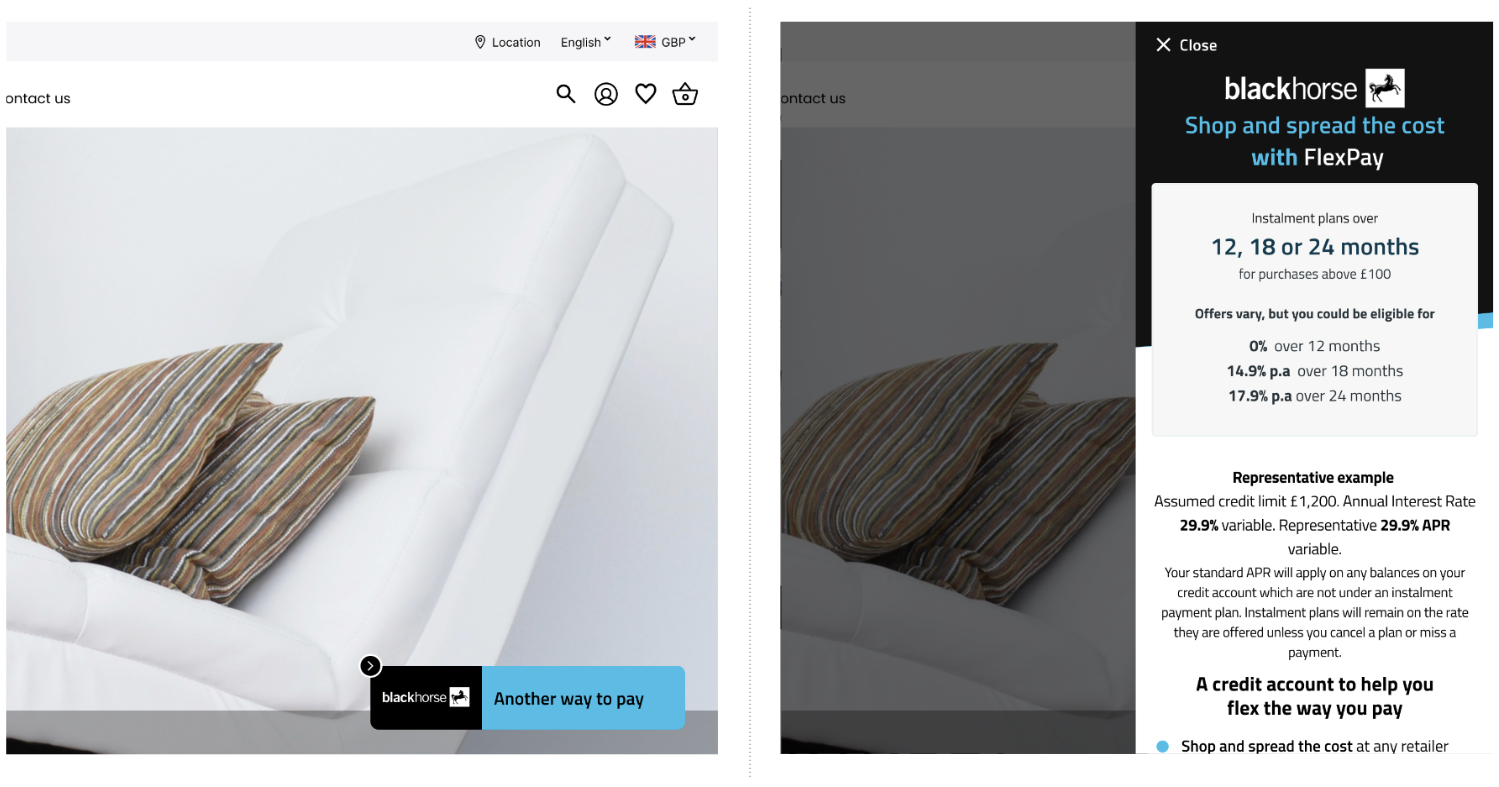
You can use the widget
Javascript object to hide or show the tile. Customers clicking on the tile outside of checkout will open the FlexPay Widget and be able to see available Finance Offers based on a standard purchase amount.
widget.hide();
widget.show();
Note: if you need to, for example it conflicts with your page layout or navigation, you can change the position of the tile using custom CSS. Find out how to do this in our tile position appendix.