FlexPay Widget is implemented using a server side FlexPay API through which you can obtain a unique access token for your given merchant, and a Javascript library which enables you to display the widget and embed finance natively in your website.
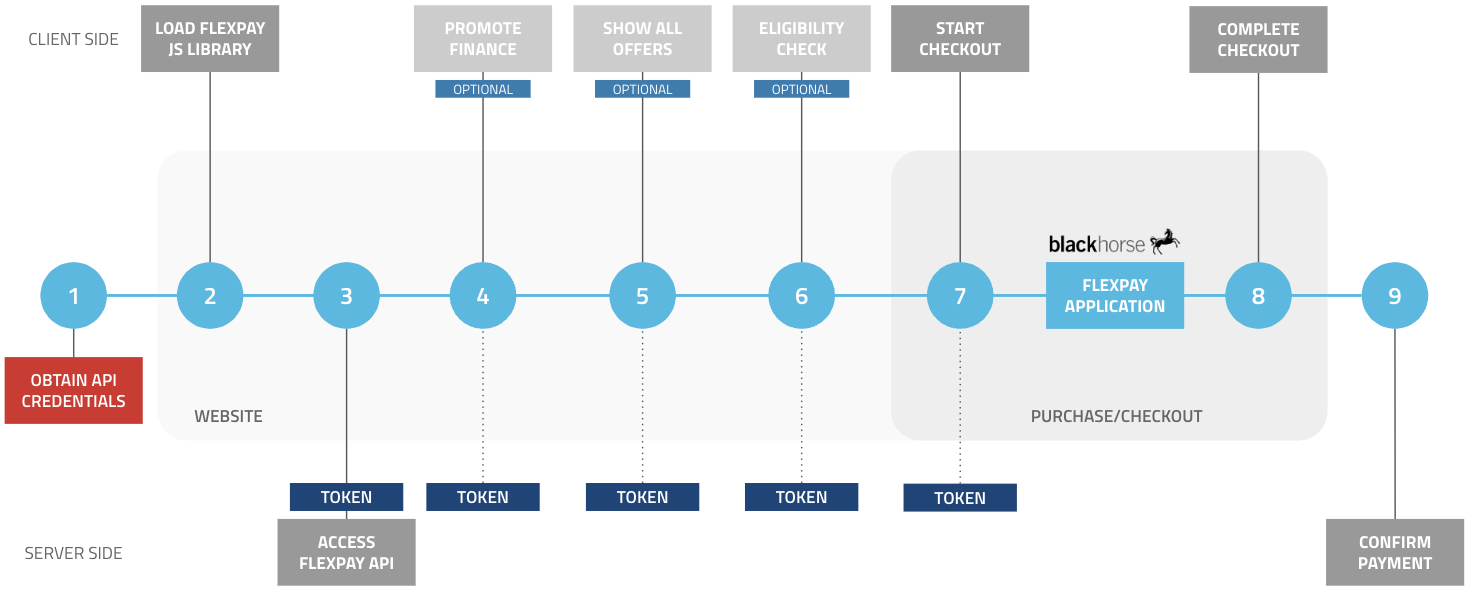
Key Steps
- Obtain API Credentials for your website
- Load the FlexPay Javascript Library on your website
- Access FlexPay API to authenticate and obtain an access token
What You'll Need | Initialise FlexPay Widget | Show Finance Offers | Eligibility Check | Start Checkout | Complete Checkout | Confirm Payment
1. Obtain API Credentials
We'll issue you a Client ID and Client Secret for your website. These are required to generate your access token for all of the steps in this section. If you have more than one website or brand then each one will have different client credentials for User Acceptance Testing (UAT) and Production (Live).
All example curl commands are to show the format of the request and response, but use a dummy API hostname, which will need to be replaced with the relevant UAT and Production hostnames. Current FlexPay API hostnames for our UAT and Live environments are available in the Environments section.
Protecting Your Credentials
It is important that you keep your credentials secure whether they are being transmitted locally in your organisation and devices, or implemented in your systems and servers. You could create a secure credential server and simple encapsulated function that creates FlexPay Widget access tokens.
2. Load FlexPay Javascript Library
The FlexPay integration requires a client-side step. You will need to load the FlexPay Javascript checkout bundle. The following script tag should be placed close to the end of the head tag in your main index file:
<script src="https://assets.blackhorseflexpay.co.uk/widget/index.js" type="application/javascript"></script>
3. Access FlexPay API
In order to prevent unauthorised access, you must authenticate all requests using an access token generated using your unique credentials. To complete authentication, send an HTTP POST request to the auth endpoint, together with your credentials as the payload: client_id and client_secret.
Server-Side Authentication
Unlike the FlexPay Javascript library, you should access the FlexPay API directly from a service executed on your server, rather than in the client browser. This ensures the security of your credentials used for authentication and the integrity of the resulting access token.
You can see a an example code snippet below:
curl -X 'POST' \
'https://dummy-api.staging.host.com/auth' \
-H 'accept: application/hal+json' \
-H 'Content-Type: application/hal+json' \
-d '{
"client_id": "MERCHANT CLIENT ID",
"client_secret": "MERCHANT CLIENT SECRET"
}'
{
"access_token": "eyJhbGciOiJSUzI1aDFd45gcCI6IkpXVCIsImtpZCI6Imp6SXZmaU1HNkJqVEpUWGxkRlVwZSJ9.eyJpc3MiOiJodHRwczovL2Rla29wYXktbWVyY2hhbnQtZGV2LmV1LmF1dGgwLmNvbS8iLCJzdWIiOiJwUUhyYkJLcnBOVG1MZ043UEpSVHpJM1JiT0hVZFY4ZEBjbGllbnRzIiwiYXVkIjoiaHR0cHM6Ly9hcGkuZGVrby11YXQuY29tIiwiaWF0IjoxNjQyNDk4MjI5LCJleHAiOjE2NDI1ODQ2MjksImF6cCI6InBRSHJiQktycE5UbUxnTjdQSlJUekkzUmJPSFVkVjhkIiwiZ3R5IjoiY2xpZW50LWNyZWRlbnRpYWxzIn0.p9tisXjwPbOyZ7xodq34-oT3jopJSn7XIOgnzNbVEBsvNHQ94njSgUhv0RCDfSDEUoMEtqJXaR-TbS09dlB8HI1DO6EFRD4BaQ6HqFqoEM0Ad8elQDrN1DnUiR-ggh2Lh2BWgqK3ke2vz_1MYg4tBRlmRU0nGb1T4fMHKPHI7kxtgR_3C6thGQ_tK22QLEx4sRAPx5-KEWkiEh_OJvt6XH851KHO4uihr0ldNm_yIMayjn34c20HMRGUC869dzPsxTc3hb5X0KZ7X3M9PKqd2IFLgoYAKZBykp9xdP4RAL4TCyUjmSzKEXw1jFXDbaaJJOdHyzS2ogTR0XjFylLo-w",
"expires_in": 84255,
"token_type": "Bearer"
}
The POST request returns an access_token
, which you should add in the Authorisation header of any subsequent API requests.
To protect our servers, this endpoint is rate limited. This auth token should be cached for the time specified in the 'expires_in
' field. This token can then be used on any further request to our API until its expiration without requesting a new auth token.
Refreshing your Access Token
Please don't forget to obtain a new access token periodically according to the
expires_in
time, using the above steps. An Access Token will not work indefinitely and your integration will cease to work once the Access Token has expired, unless it has been replaced.