What You'll Need | Initialise FlexPay Widget | Show Finance Offers | Eligibility Check | Start Checkout | Complete Checkout | Confirm Payment
7. Start Checkout
If the FlexPay Widget is initialised, regardless of whether you chose to show finance offers throughout your site, then at checkout you can initiate a finance sale by providing FlexPay details of the proposed purchase. This is done securely via API in order to obtain an additional authenticated JSON Web Token (JWT).
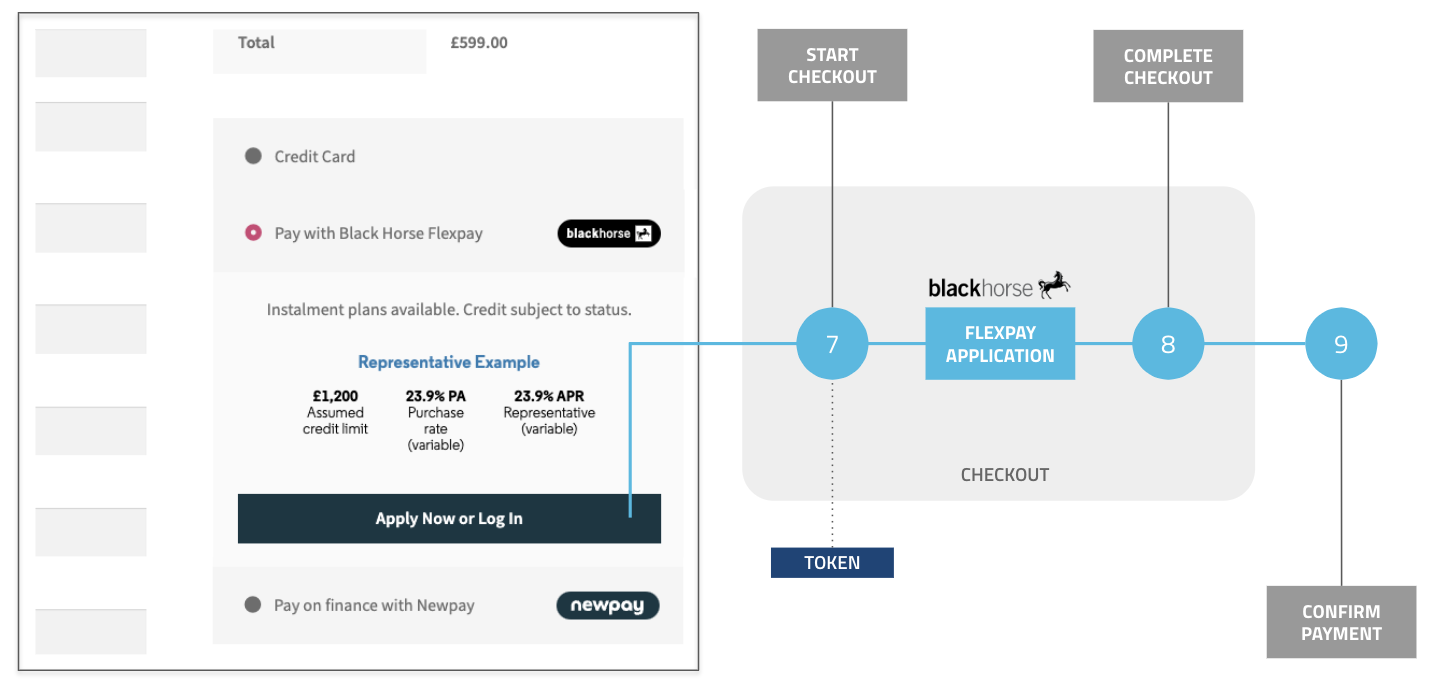
Depending on your website presents payment options, you may initiate FlexPay checkout via a button press, form submission or other action. Traditional eCommerce platforms often use a single HTML form at checkout that changes the form action and submit button text based on the selected payment option.
Notice in the above example that there is also a payment option for the secondary lender, NewDay. This is because if a customer is not eligible for Black Horse FlexPay, they may be able to choose to check their eligibility for Newpay or they could be a returning customer who has previously been approved for Newpay - so you will need to include both options.
You can view further details of the API schema in the API Reference
Submit Purchase
You can start a purchase in your website with FlexPay by submitting details to us of the customer and their proposed purchase. To eliminate the risk of customer or purchase data being intercepted or tampered with, this is done privately via API to a verify-basket
endpoint which returns an additional JSON Web access Token (JWT).
This token confirms to the FlexPay Widget that the intended purchase is valid and authenticated, enabling the Widget to launch the FlexPay finance application and purchase journey within your site. A request to the verify-basket
endpoint would typically include the following objects as detailed in the full API Reference:
customer
- personal details of the customer to perform the finance applicationbasket
- total value of the purchase and a breakdown of each individual line item*reference
- a unique reference from your platform to easily reconcile the purchaseproducts
- an array containing the product to checkout with, in this caseblackhorse-flexpay
callbackUri
- a script URL on your server where Flexpay can provide status updates
In response, you will receive an additional JWT.
curl -X 'POST' \
'https://dummy-api.staging.host.com/verify-basket' \
-H 'accept: application/hal+json' \
-H 'Authorization: Bearer xeyJhbGciOiJSUzI1NiIsInR5cCI6IkpXVCIsImtpZCI6Imp6SXZmaU1HNkJqVEpUWGxkRlVwZSJ9.eyJpc3MiOiJodHRwczovL2Rla29wYXktbWVyY2hhbnQtZGV2LmV1LmF1dGgwLmNvbS8iLCJzdWIiOiJ2TDBac0VOOWo1TlQ0VlAzY0ZLQnAxakY5NHNtNUM4dkBjbGllbnRzIiwiYXVkIjoiaHR0cDovL2xvY2FsaG9zdDo4MDgxL2FwaS9jaGVja291dCIsImlhdCI6MTYxNjU5MTU4NywiZXhwIjoxNjE2Njc3OTg3LCJhenAiOiJ2TDBac0VOOWo1TlQ0VlAzY0ZLQnAxakY5NHNtNUM4diIsImd0eSI6ImNsaWVudC1jcmVkZW50aWFscyJ9.F-dzPkJVF0ub2kGiWn1As5PSMnIiqAriOhyux47t7lbNk0tD9Wu655JGHmjuAqqQ3uWyqXx9-feuYjv61xufgBfxH17OkIqBB9lVJzEONoVqF5uIzsnaTD2z0FTzcvOuH8RXzA-_q7vFZwvbboE9iZCFd-JN8vI_1jjWDUpdKFWzGk0-AmID9F7qgNnyC2WEb6DK4Ky5VttXPXcGfnIGD2ybOgkg1dOlBhzabJBT9jU_UQuBoKjqQoNqVm1ovhFJzzSWMsHqDIDOb7r-0zXRB33ka4_oKoVnVrvxL2hGblgC-2Jg6gfB7uuVPdbM5FIBFx8Cbt4T83KxXbpJy5hQbg' \
-H 'Content-Type: application/json' \
-d '{
"customer": {
"billingAddress": {
"title": "Dr",
"firstName": "Jane",
"lastName": "Doe",
"middleName": "R.",
"address1": "Deko",
"address2": "15 Bishopsgate",
"town": "Cornhill",
"county": "London",
"postcode": "EC2N 3AR",
"country": "GB"
},
"shippingAddress": {
"title": "Dr",
"firstName": "Jane",
"lastName": "Doe",
"middleName": "R.",
"address1": "Deko",
"address2": "15 Bishopsgate",
"town": "Cornhill",
"county": "London",
"postcode": "EC2N 3AR",
"country": "GB"
},
"phone": "00447000123456",
"email": "[email protected]",
"birthDate": "1996-07-01"
},
"basket": {
"currency": "GBP",
"total": 134523,
"items": [
{
"description": "A shiny gold watch",
"quantity": 1,
"price": 134523,
"type": "sku",
"imageUri": "https://your-platform.fake/product/image/uri.jpg",
"itemUri": "https://your-platform.fake/product1.html"
}
]
},
"products": [
"blackhorse-flexpay"
],
"reference": "d290f1ee-6c54-4b01-90e6-d701748f0851",
"callbackUri": "https://your-platform.fake/callback"
}'
Launch FlexPay
While browsing finance offers in the Widget is possible anonymously outside checkout without calling this API, for starting and completing a checkout purchase it is necessary first to obtain the additional JWT access token as above. You can then use widget.checkout()
to trigger the transaction process.
The function you pass to the flexpayWidget.checkout
method must make a request to your server which in turn should request the checkout access token from the FlexPay API. The return type of your function should be a Promise that resolves to the JWT token retrieved by your server, similar to the init
method.
const yourCheckoutFunction = () => {
return Promise.resolve(myCheckoutToken);
}
// Note using the wallet instance created in the Initialise step above.
widget.checkout(yourCheckoutFunction);
Previously we explained how the Widget allows you to show and hide the promotional tile. You can also use the same Javascript commands to manually open and close the wallet before or during a transaction:
widget.close();
widget.open();